Javascript Currying
— Javascript, Vanilla, Function — 1 min read
Currying is advanced technique of working with functions. Actually this concept come from lambda calculus. It's used not only in JavaScript, but in other languages as well. So in this blog I'll explain in JavaScript.
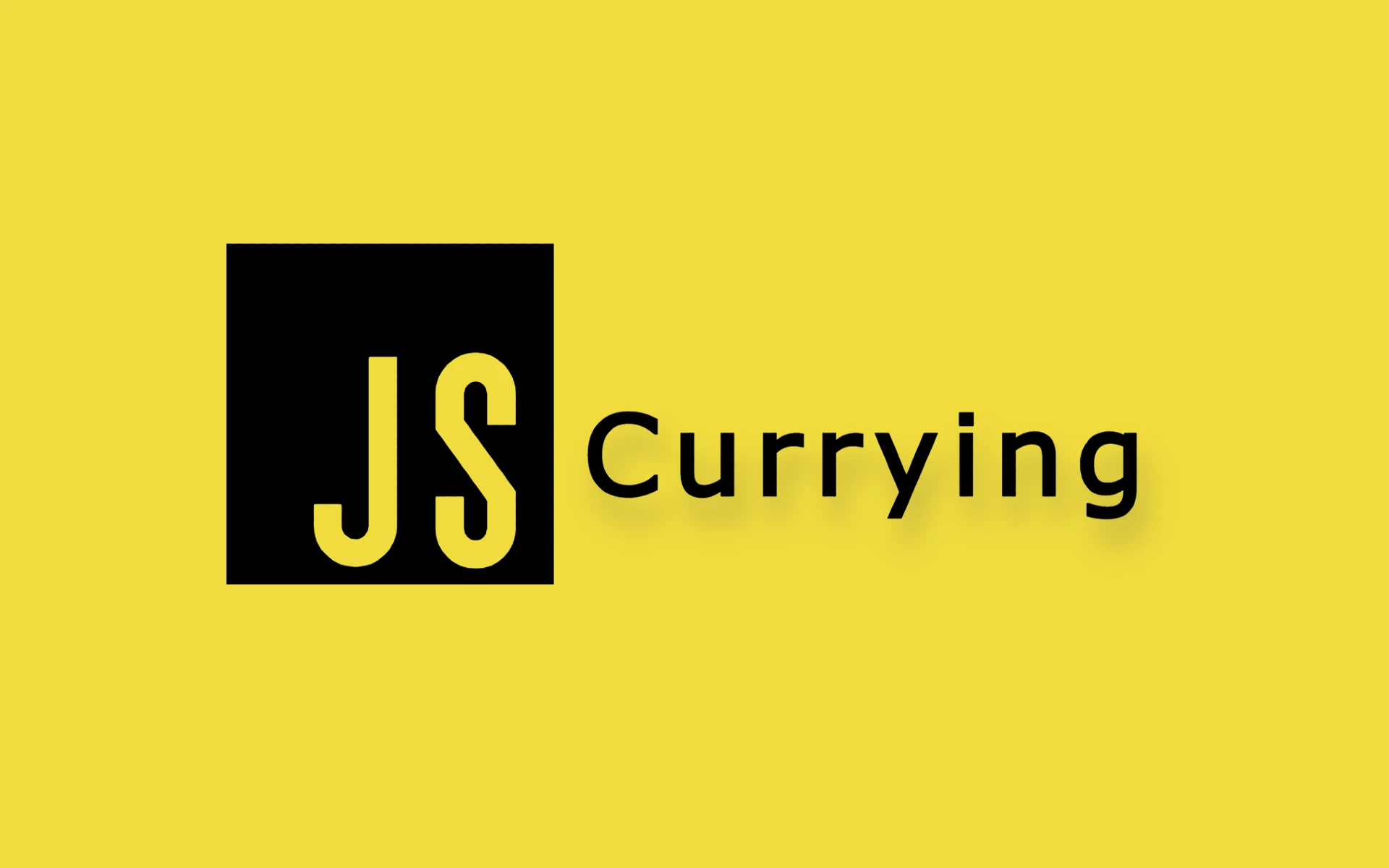
Currying doesn't call a function. It just transforms it. Let's say you have f(a,b,c)
function it takes many parameters. Curried function transform the function to pieces for example f
function => f(a)(b)(c)
. So, to better understand lets make an example.
1// Let's say you have a fetcher function to make a API call2function fetcher(url, options) {3 return fetch(url, options);4}5// For each method you should pass a parameter to what kind of http method want to use6// This is not effective way to use a function when you learn currying7// Without curring8const data = fetcher('url.com', 'GET');9const data = fetcher('url.com', 'POST');10
11/*-------------------------------------*/12//13function curriedFetcher(options) {14 return function (url) {15 return fetch(url, option);16 };17}18
19// Usage20curriedFetcher('GET')('url.com');21curriedFetcher('POST')('url.com');22curriedFetcher('POST', 'url.com'); // Don't Work!23
24// With arrow function25const curriedFetcherArrow = (options) => (url) => fetch(url, option);26
27const getRequest = curriedFetcherArrow('GET');28const postRequest = curriedFetcherArrow('POST');29// Usage30getRequest('url.com');31postRequest('url.com');
As you can see, the implementation is quiete easy. Also in line 19 something you might be use it case of some how. But it doesn't work like that. You might use a library for it. The common utils library lodash also has a curry function to transform functions to curried functions. And also you can work with previous version of function. That will be good if you need it. Let's checkout how can implement a library for it.
Conclusion
In this blog we learned what is currying and how to implement it. Also we learned how to use it with lodash. I hope you enjoyed it. If you have any questions or suggestions please reach me with links on top of the page. Thanks for reading.