What the heck is a "memoization"?
— Javascript, memoization, advanced — 1 min read
Memoization is a technique to cache the results of a function. It's a way to speed up your code. It's a way to avoid unnecessary calculations. It does this by storing computation results in cache, and retrieving that same information from the cache the next time it's needed instead of computing it again.
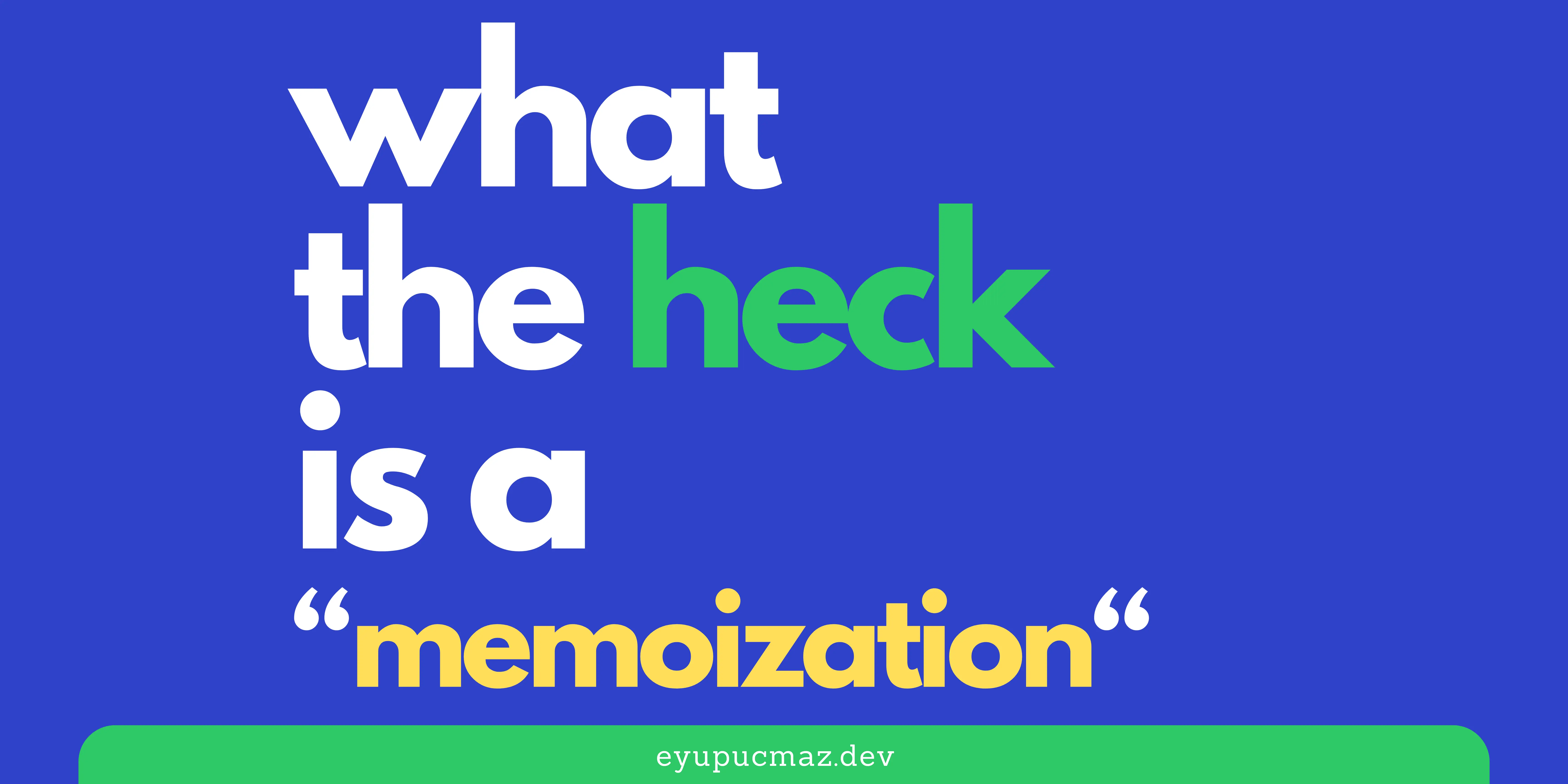
For better understanding, let's take a look at an example.
1function recursiveFib(order) {2 if (order < 2) {3 return order;4 }5
6 return recursiveFib(order - 1) + recursiveFib(order - 2);7}
The function above is a recursive function that calculates the Fibonacci sequence. It's a sequence of numbers where each number is the sum of the two preceding ones, starting from 0 and 1.
1function memoFib(num: number) {2 if (!memoFib.cache) {3 memoFib.cache = {};4 }5 if (memoFib.cache[num]) {6 return memoFib.cache[num];7 }8 if (num < 2) return num;9
10 memoFib.cache[num] = memoFib(num - 1) + memoFib(num - 2);11 return memoFib.cache[num];12}
The function above is a memoized version of the recursive function above. It's a function that caches the results of a function. Javascript functions are object and can have properties. The property cache
is used to store the results of the function. The function checks if the result is already in the cache, if it is, it returns the result from the cache. If it's not, it calculates the result and stores it in the cache.
Lets see how the performance of the two functions compare.
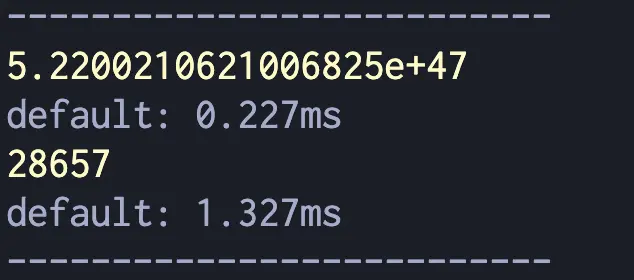
First calculation is log of console.log(memoFib(230))
and it takes 0.227 ms
. Second calculation is log of console.log(recursiveFib(23))
and it takes 1.327 ms
. That's a huge difference. The first calculation is much faster because it's not calculating the result again, it's just retrieving it from the cache. Also attention the difference between the two input values. The first one is 230
and the second one is 23
. The first one is 10
times bigger than the second one. But the first calculation is 6
times faster than the second one. That's because of memoization.
Conclusion
This technique is very useful when you have a function that takes a lot of time to calculate the result. You should use it when you have a function that takes a lot of time to calculate the result and you need to call that function multiple times with the same input values. If you have a function that takes a lot of time to calculate the result and you need to call that function only once, you don't need to use memoization. You can just call the function once and store the result in a variable. It will be faster than memoization.